本文将介绍如何快速完成 TUICallKit 组件的接入,您将在10分钟内完成以下几个关键步骤,并最终得到一个包含完备 UI 界面的视频通话功能。
视频通话 | 语音通话 |
![]() | ![]() |
单人通话 | 群组通话 |
![]() | ![]() |
TUICallKit Demo 体验
如果您想要直接体验音视频通话,进入 Demo 体验页面,点击 Web 卡片。
如果您想要直接跑通一个新工程,请直接阅读 Web Demo 快速跑通。
环境准备
Node.js 版本:Node.js ≥ 12.13.0(推荐使用官方 LTS 版本,npm 版本请与 node 版本匹配)。
Modern browser, supporting WebRTC APIs.
webpack 版本:webpack ≥ 5.0.0。
npm 包集成
Vue3 开发环境,集成 @tencentcloud/call-uikit-vue NPM 包。
Vue2.7 开发环境:集成 @tencentcloud/call-uikit-vue2 NPM 包。
Vue2.6 开发环境:集成 @tencentcloud/call-uikit-vue2.6 + @vue/composition-api NPM 包。
源码集成
Vue3 + TypeScript 开发环境:从 @tencentcloud/call-uikit-vue NPM 包拷贝源码。
Vue2.7 + TypeScript 开发环境:从 @tencentcloud/call-uikit-vue2 NPM 包拷贝源码。
Vue2.6 + TypeScript 开发环境:从 @tencentcloud/call-uikit-vue2.6 NPM 包拷贝源码。
需要:Vue2.6 + unplugin-vue2-script-setup + @vue/composition-api 。
注意:
1. HBuilderX 中选择 vue2 时,使用的是 vue2.6,因此需要使用:@tencentcloud/call-uikit-vue2.6。
2. HBuilderX 中选择 vue3 时,使用的是 vue3,因此需要使用:@tencentcloud/call-uikit-vue。
步骤一:开通服务
步骤二:创建 Vue3 项目
1. 如果您尚未安装 vue-cli ,可以在 terminal 或 cmd 中采用如下方式进行安装:
npm install -g @vue/cli
2. 通过 vue-cli 创建项目,并选择下图中所选配置项。
vue create tuicallkit-demo
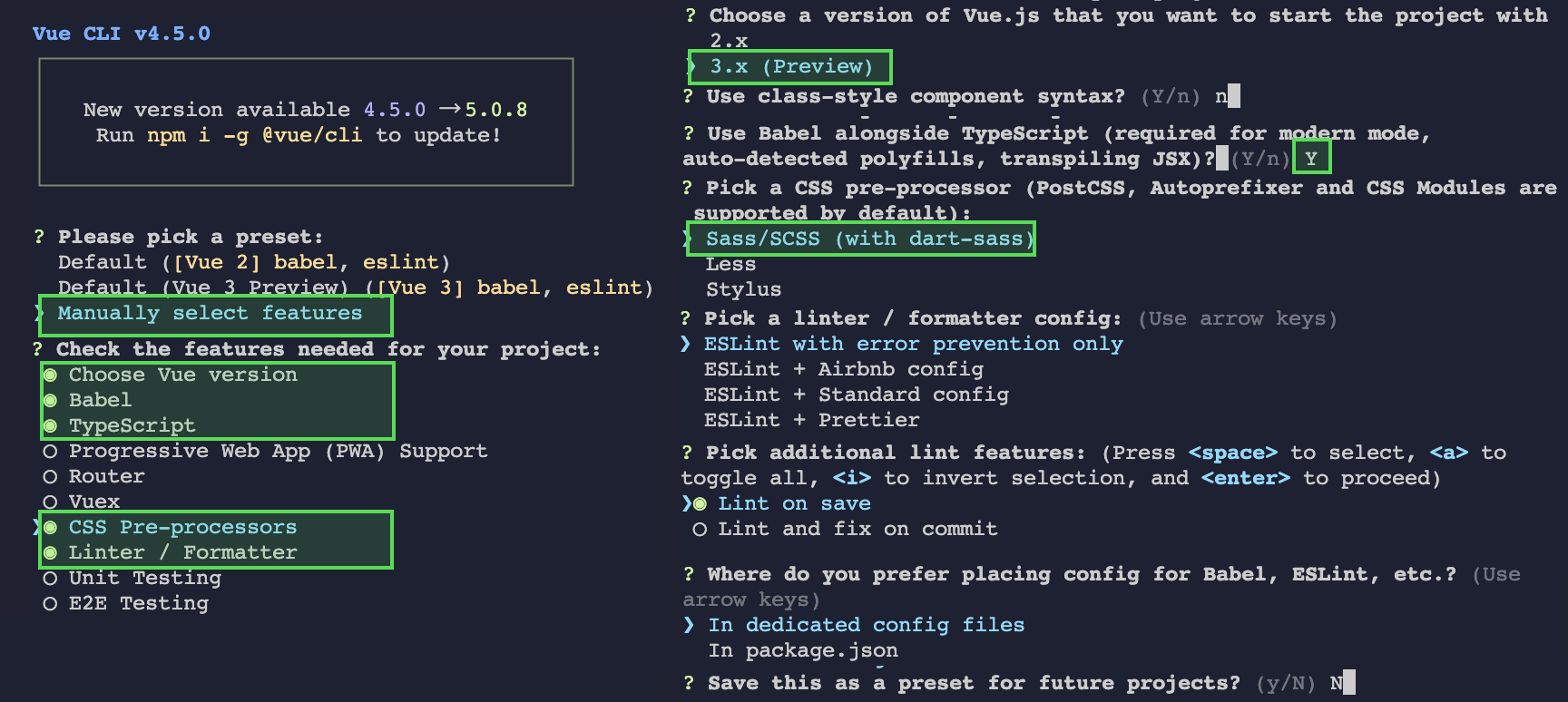
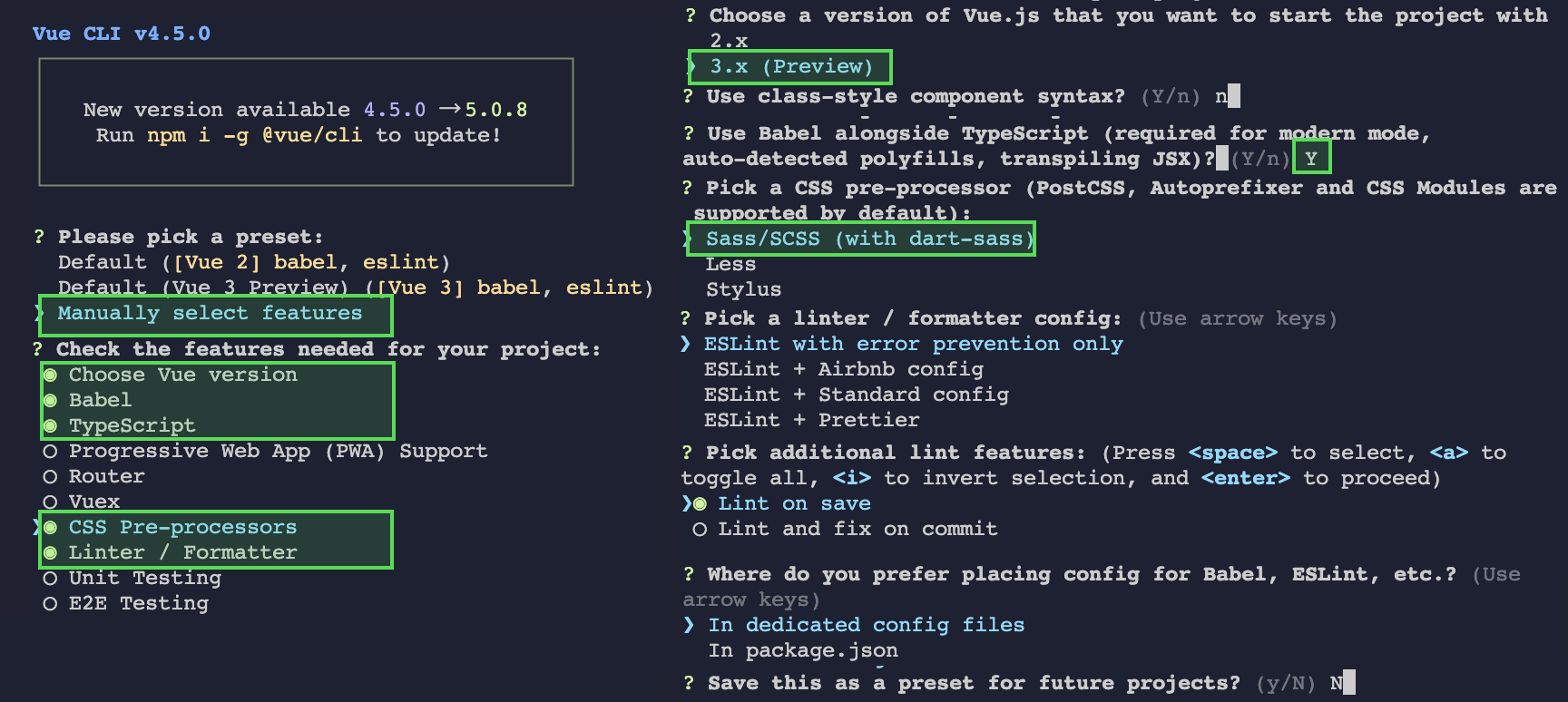
3. 创建完成项目,切换到项目所在目录。
cd tuicallkit-demo
步骤三:下载 TUICallKit 组件
1. 通过 npm 方式下载 @tencentcloud/call-uikit-vue 组件并在项目中使用。
npm install @tencentcloud/call-uikit-vue
npm install @tencentcloud/call-uikit-vue2
npm install @tencentcloud/call-uikit-vue2.6 @vue/composition-api
npm i -D unplugin-vue2-script-setup
2. 将 debug 目录复制到您的项目。
cp -r node_modules/@tencentcloud/call-uikit-vue/debug ./src
xcopy node_modules\\@tencentcloud\\call-uikit-vue\\debug .\\src\\debug /i /e
cp -r node_modules/@tencentcloud/call-uikit-vue2/debug ./src
xcopy node_modules\\@tencentcloud\\call-uikit-vue2\\debug .\\src\\debug /i /e
cp -r node_modules/@tencentcloud/call-uikit-vue2.6/debug ./src
xcopy node_modules\\@tencentcloud\\call-uikit-vue2.6\\debug .\\src\\debug /i /e
步骤四:引入 TUICallKit 组件
1. 如下代码示例中的 SDKAppID、SecretKey 、userID、callUserID 四个参数。
SDKAppID:在腾讯云创建的音视频应用 SDKAppID,参见 开通服务。
SecretKey:用户签名,参见 开通服务。
userID:主叫的 userID,字符串类型,只允许包含英文字母(a-z 和 A-Z)、数字(0-9)、连词符(-)和下划线(_)。
callUserID:被叫的 userID,需要已初始化存在。(demo 中的 callUserID,当有被叫时输入,无被叫可以不输入)。
2. 在
tuicallkit-demo/src/App.vue
中引入下面代码。说明:TUICallKit 组件需要放到一个 dom 节点里,用于显示并控制 TUICallKit 的位置、宽高等样式。
将下面代码直接复制引用在
App.vue
文件中。<template><div style="padding: 20px 0 0 20px"><div><span>userID:</span><input type="text" v-model="userID" /></div><div style="margin-top: 6px;"><span>callUserID:</span><input type="text" v-model="callUserID" /></div><button @click="init()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 1: Initialization </button><button @click="call()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 2: Start 1v1 video call </button><div style="width: 50rem; height: 35rem; border: 1px solid salmon;"><TUICallKit /></div></div></template><script lang="ts" setup>import { ref } from 'vue';import { TUICallKit, TUICallKitServer, TUICallType } from "@tencentcloud/call-uikit-vue";import * as GenerateTestUserSig from "./debug/GenerateTestUserSig-es";const userID = ref('');const callUserID = ref('');const SDKAppID = 0; // Replace with your SDKAppIDconst SecretKey = ''; // Replace with your SecretKeyasync function init() {try {const { userSig } = GenerateTestUserSig.genTestUserSig({userID: userID.value,SDKAppID: SDKAppID,SecretKey: SecretKey,});await TUICallKitServer.init({ //【1】Initialize the TUICallKit componentSDKAppID,userID: userID.value,userSig,});alert("[TUICallKit] Initialization success.");} catch (error: any) {alert(`[TUICallKit] Initialization failed. Reason: ${error}`);}}async function call() { //【2】Make a 1v1 video calltry {await TUICallKitServer.call({ userID: callUserID.value, type: TUICallType.VIDEO_CALL });} catch (error: any) {alert(`[TUICallKit] Call failed. Reason: ${error}`);}}</script>
将下面代码直接复制引用在
App.vue
文件中。<template><div style="padding: 20px 0 0 20px"><div><span>userID:</span><input type="text" v-model="userID" /></div><div style="margin-top: 6px;"><span>callUserID:</span><input type="text" v-model="callUserID" /></div><button @click="init()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 1: Initialization </button><button @click="call()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 2: Start 1v1 video call </button><div style="width: 50rem; height: 35rem; border: 1px solid salmon;"><TUICallKit /></div></div></template><script>import { TUICallKit, TUICallKitServer, TUICallType } from "@tencentcloud/call-uikit-vue2";import * as GenerateTestUserSig from "./debug/GenerateTestUserSig-es";export default {name: 'App',data() {return {// SDKAppID、userSig 的获取参考下面步骤userID: '', // 主叫的 userIDcallUserID: '', // 被叫的 userIDSDKAppID: 0, // Replace with your SDKAppIDSecretKey: '', // Replace with your SecretKey};},components: {TUICallKit},methods: {async init() {try {const { userSig } = GenerateTestUserSig.genTestUserSig({userID: this.userID,SDKAppID: Number(this.SDKAppID),SecretKey: this.SecretKey,});// 初始化await TUICallKitServer.init({SDKAppID: Number(this.SDKAppID),userID: this.userID,userSig,// tim: this.tim // 如果工程中已有 tim 实例,需在此处传入});alert("[TUICallKit] Initialization succeeds.");} catch (error) {alert(`[TUICallKit] Initialization failed. Reason: ${error}`);}},async call() {try {// 1v1 video callawait TUICallKitServer.call({ userID: this.callUserID, type: TUICallType.VIDEO_CALL });} catch (error) {alert(`[TUICallKit] Call failed. Reason: ${error}`);}}},}</script>
1.
main.ts 文件
注册 @vue/composition-api 。import Vue from 'vue'import VueCompositionAPI from '@vue/composition-api'Vue.use(VueCompositionAPI)
2. 将下面代码直接复制引用在
App.vue
文件中。<template><div style="padding: 20px 0 0 20px"><div><span>userID:</span><input type="text" v-model="userID" /></div><div style="margin-top: 6px;"><span>callUserID:</span><input type="text" v-model="callUserID" /></div><button @click="init()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 1: Initialization </button><button @click="call()" style="margin: 10px 10px 10px 0; height: 28px;"> Step 2: Start 1v1 video call </button><div style="width: 50rem; height: 35rem; border: 1px solid salmon;"><TUICallKit /></div></div></template><script>import { TUICallKit, TUICallKitServer, TUICallType } from "@tencentcloud/call-uikit-vue2.6";import * as GenerateTestUserSig from "./debug/GenerateTestUserSig-es";export default {name: 'App',data() {return {// SDKAppID、userSig 的获取参考下面步骤userID: '', // 主叫的 userIDcallUserID: '', // 被叫的 userIDSDKAppID: 0, // Replace with your SDKAppIDSecretKey: '', // Replace with your SecretKey};},components: {TUICallKit},methods: {async init() {try {const { userSig } = GenerateTestUserSig.genTestUserSig({userID: this.userID,SDKAppID: Number(this.SDKAppID),SecretKey: this.SecretKey,});await TUICallKitServer.init({SDKAppID: Number(this.SDKAppID),userID: this.userID,userSig,// tim: this.tim // 如果工程中已有 tim 实例,需在此处传入});alert("[TUICallKit] Initialization succeeds.");} catch (error) {alert(`[TUICallKit] Initialization failed. Reason: ${error}`);}},async call() {try {// 1v1 video callawait TUICallKitServer.call({ userID: this.callUserID, type: TUICallType.VIDEO_CALL });} catch (error) {alert(`[TUICallKit] Call failed. Reason: ${error}`);}}},}</script>
步骤五:拨打您的第一通电话
1. 在终端输
npm run serve
运行 tuicallkit-demo。2. 点击
Step 1: Initialization
Button,弹出 Initialization success 表示初始化成功。

3. 点击
Step 2: Start 1v1 video call
发起 1v1 视频通话,具体效果如下图所示。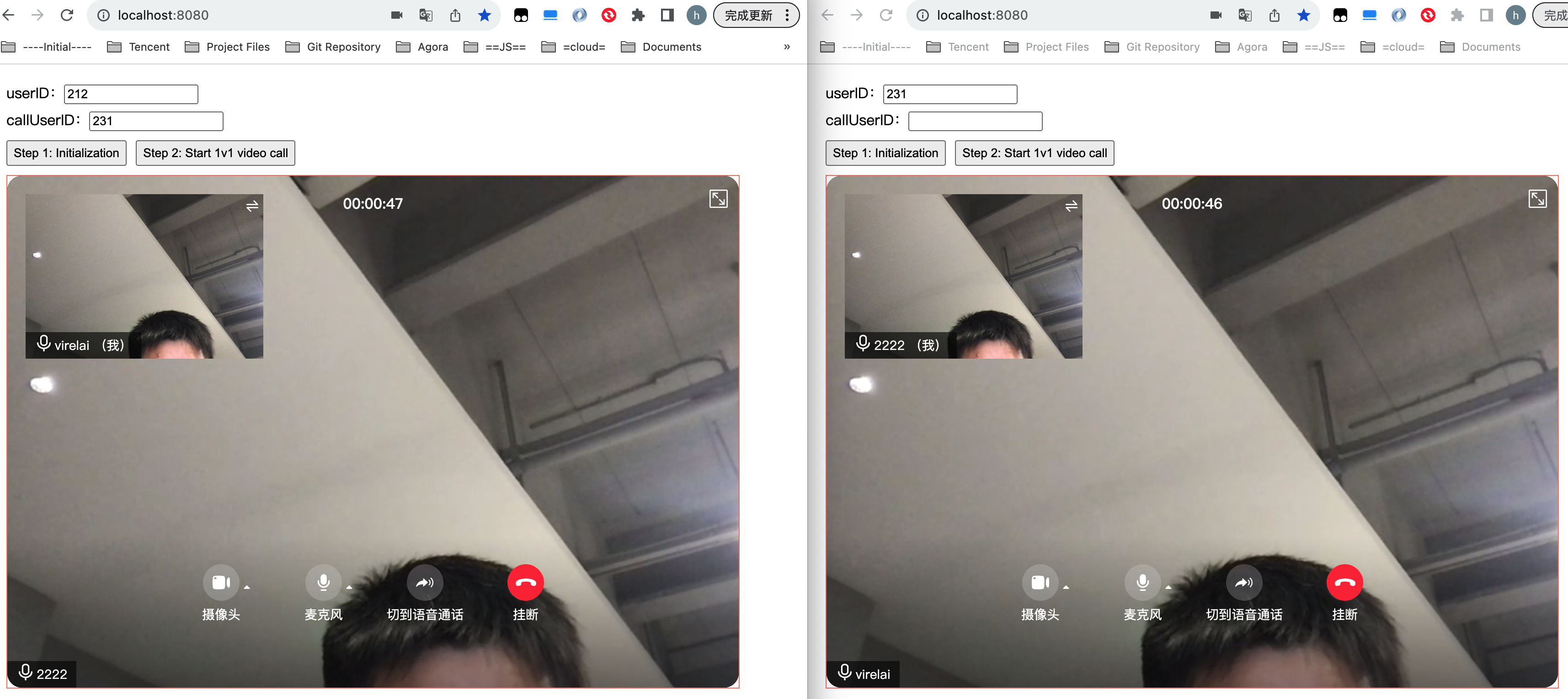
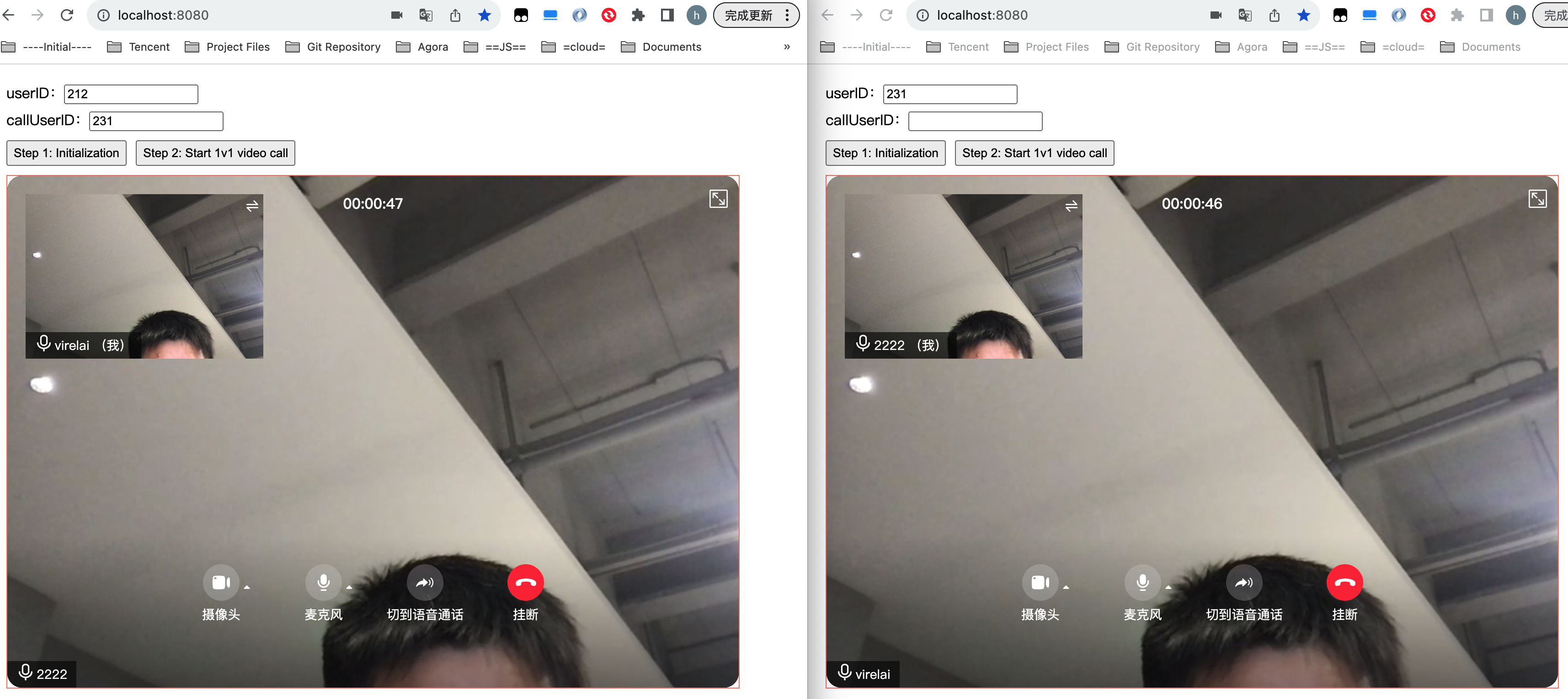
更多特性
设置昵称、头像
群组通话
悬浮窗
自定义铃声
界面定制